[Code Challenge] Missing Numbers
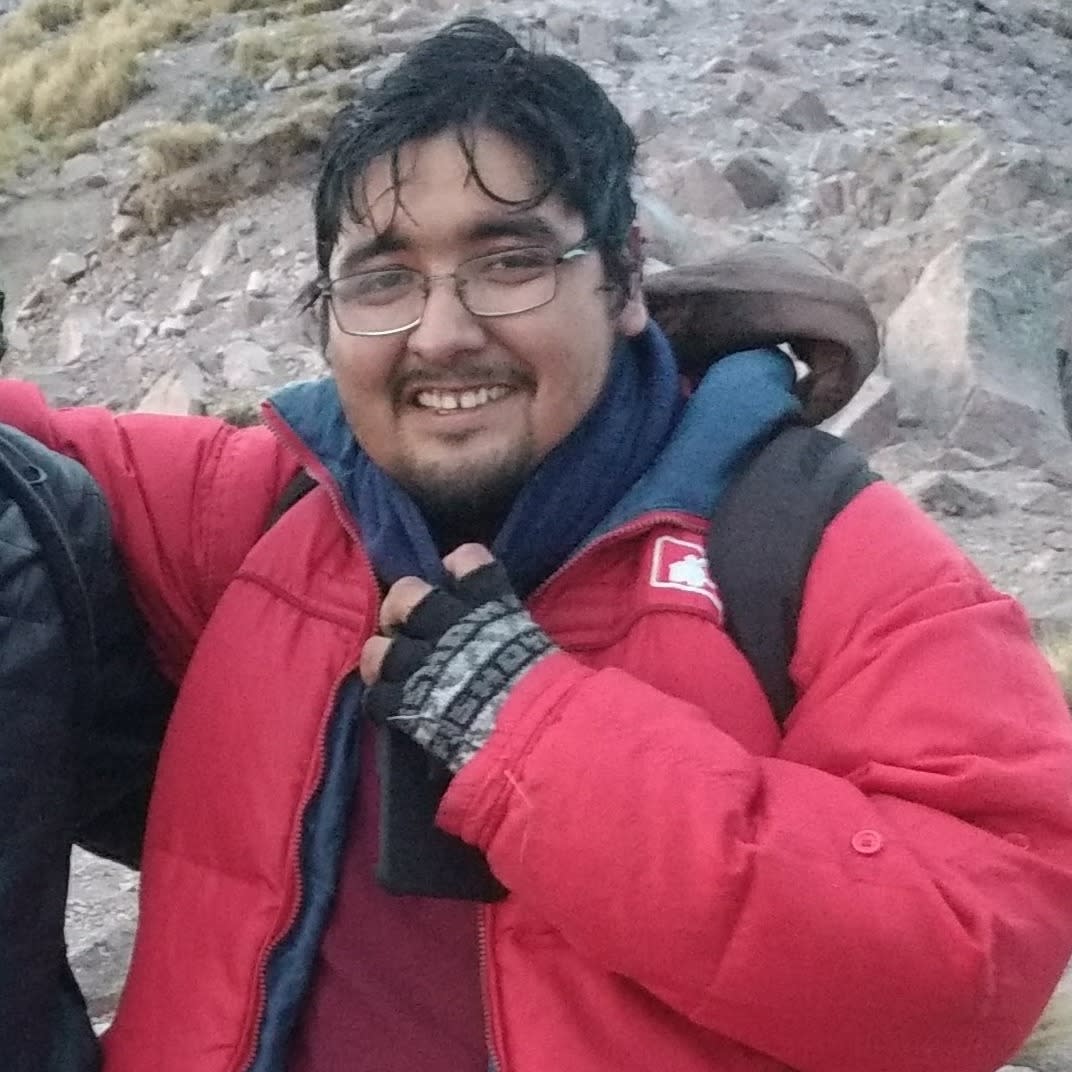
![Cover Image for [Code Challenge] Missing Numbers](http://images.ctfassets.net/l3mfryzykpa5/3wWAEtiPhA5rVgae6gYLuI/868e2f7cf686384453a2f9c9d6b0484f/code-1839406_1280.jpg?w=3840&q=75)
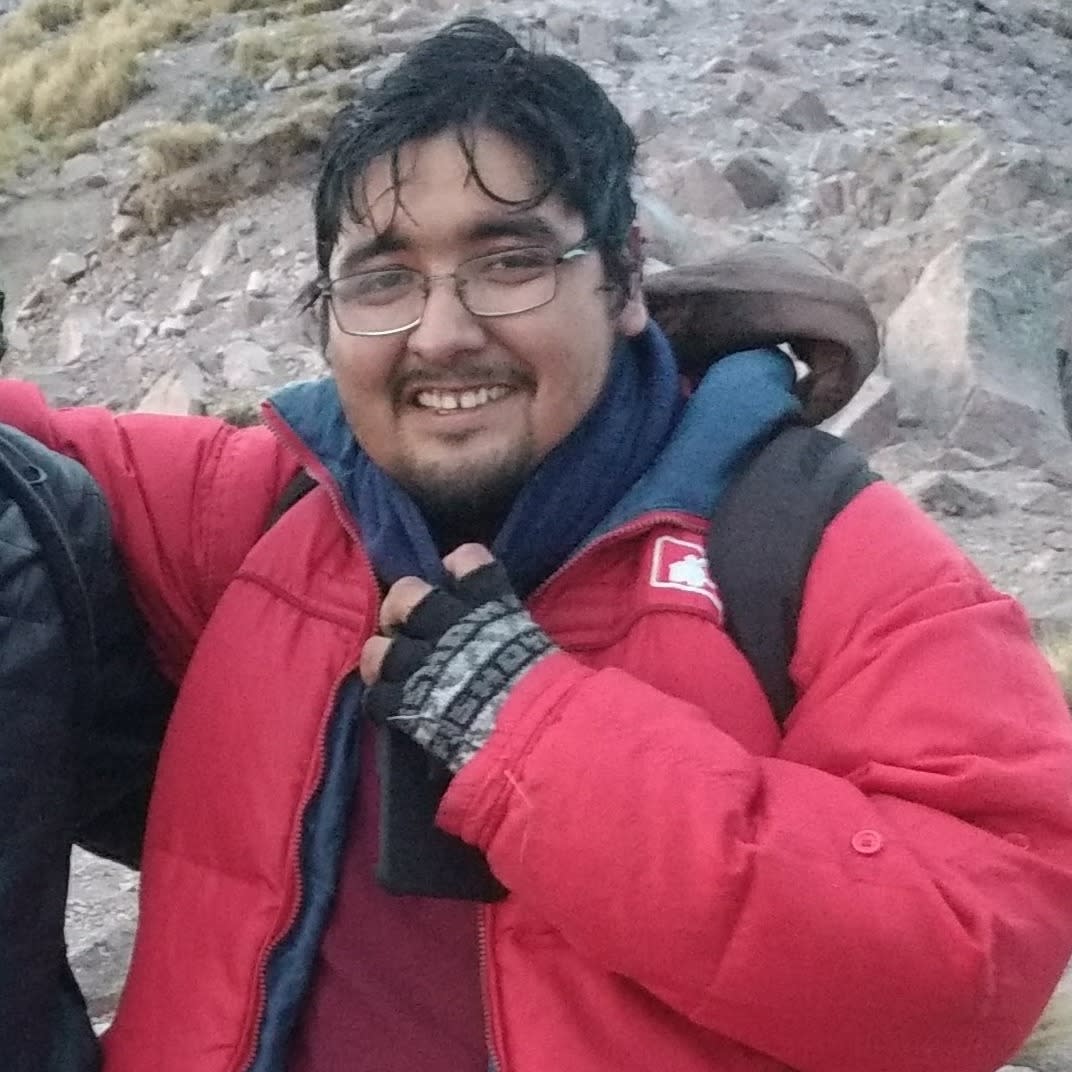
The first thing we need to do is to have in mind what is our expected outcome. The problem stattes that we will always have an array of integers of length "n" and we will end with a similar array but with a length of "n+2".
Also, the problem states that all the numbers are integers and we always start from 1 up to n. So, we can say that we can know what is the expected amount from the begining. Think about it, if we will always have an array of incremental numbers, we can calculate the sum of all the expected elements and then find this missing numbers.
Follow me on this. Imagine that you have an array like [1, 4, 3], you know that your final array will look like [1, 2, 3, 4, 5], because we will always need 2 numbers no matter what. So, in our first array our summatory will look like 1+3+4=8. And our expected summatory will be 1+2+3+4+5=15, The we can say that 15-8=7. And then we can state that we need 2 positive integers, one greater than the other, and when added they give us an amount of 7.}
We do that first by caculating the average of this number and we will look at the bottom part. So, if we know that our number is 7. Then 7/2 = 3.5, but we need to rounded to the floor, so 3 will do the trick. Then we need to validate if this number is missing in the array. In the case it is, then we push it into the array, negative case we decrease it by 1 and compare it again until we find the missing one.
At the end we will have an array of 4 elements, in this case we can repeat the initial process, we just can just substract the sum of all the current elements from the expected amount, and then we will have all our missing parts.
function missingNumbers(nums) {
let res = [];
let targetSum = 0;
for(let i=1; i<=nums.length+2; i++){
targetSum+=i;
} let currentSum = nums.reduce((sum, current) => sum + current, 0);
let difference = targetSum - currentSum;
let minNum = Math.floor(difference/2);
while(minNum>0){
if(!nums.includes(minNum)){
res.push(minNum);
nums.push(minNum);
minNum = 0;
}
else{
minNum--;
}
} currentSum = nums.reduce((sum, current) => sum + current, 0);
res.push(targetSum - currentSum);
return res;}